Simple Game - KIT207
In this section, I created a basic game by using the assets and concepts from the previous dev logs.
So the first thing I did was to import the KIT207 Assets which included the following scripts.
- PlayerHealth.cs - What it says, It's the player health.
- Spawner.cs - Enemy Spawner.
- TriggerDetection.cs - When a trigger enter or exit is detected, it fires a unity event which I can assign directly in unity.
So the first thing I did was add the PlayerHealth script to the player GameObject and create a Text GameObject by going to GameObject > UI > Legacy > Text and positioning it to the top left corner. Then I linked the Text GameObject to the PlayerHealth by dragging and dropping the Text GameObject into the Health Text variable. (I could've used TMPro but it was just easier to use the legacy one.)
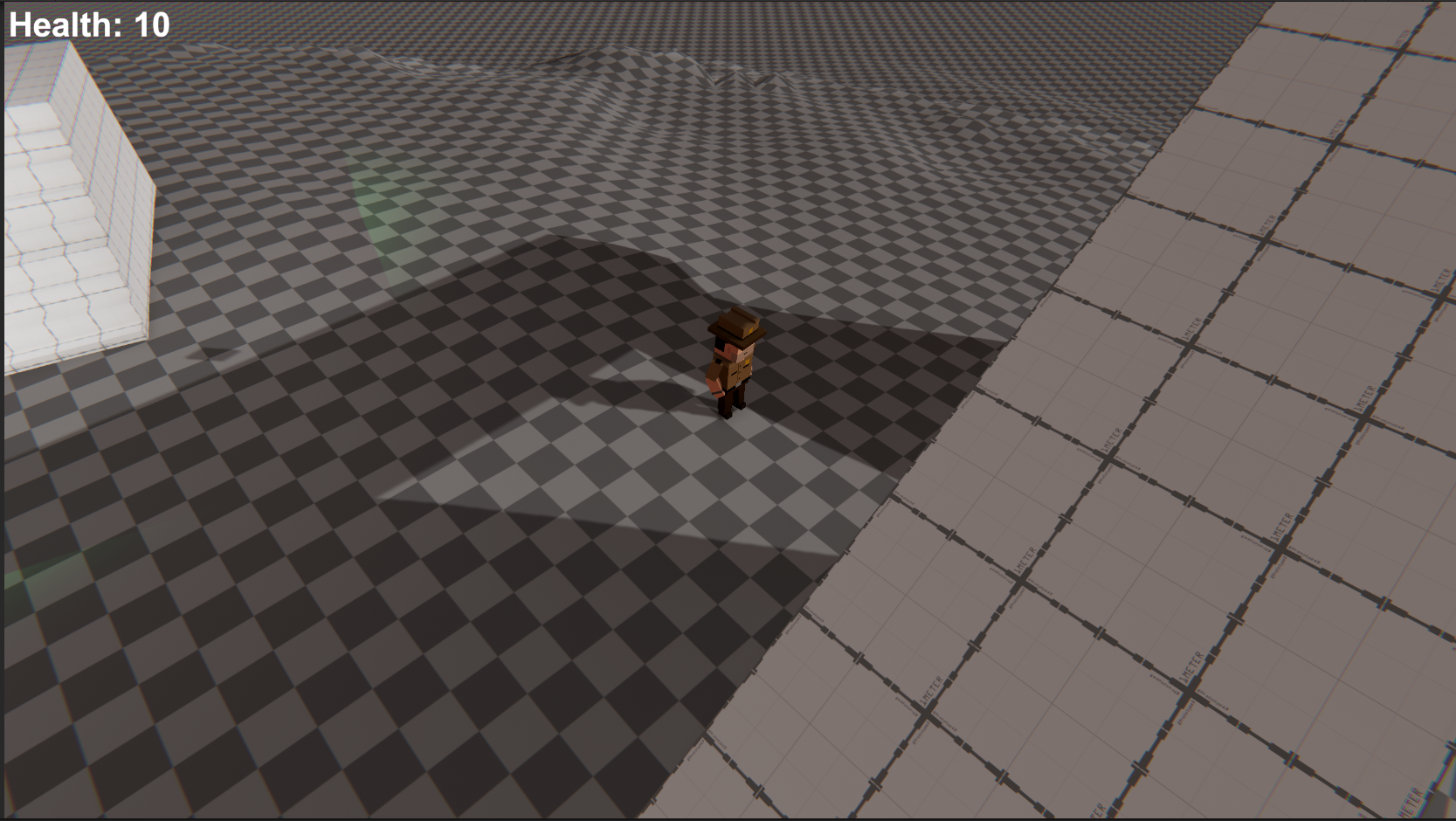
After that, I created an empty GameObject and named it Spawner. Next, I added the Spawner script to the Spawner GameObject, Set the Prefab To Spawn to the enemy prefab by dragging and dropping the enemy prefab into the variable, then I set the Interval In Seconds to 5 so an enemy would spawn every 5 seconds. However this did break my current enemy script so I changed by adding a Sphere Collider trigger onto the enemy prefab and modifying the code so the enemy script would only listen for player trigger collisions to set it's target.
private void OnTriggerEnter(Collider other)
{
if(other.CompareTag("Player"))
target = other.transform;
}
private void OnTriggerExit(Collider other)
{
if(other.transform == target)
target = null;
}
Then I added a Capsule Collider and Rigid Body for the actual collisions and added an extra piece of code onto the enemy so it would decrease the player health and destroy itself.
private void OnCollisionEnter(Collision collision)
{
if (collision.transform == target)
{
Destroy(gameObject);
collision.gameObject.GetComponent<playerhealth>().DecreaseHealth(1);
}
}
Results...
Next was adding health packs, This was fairly simple as all I had to do was use a prefab health pack from Simple Items by Synty Studios, add the TriggerDetection script and a BoxCollider to finish the setup, Then the next thing I had to do was link up the event by pressing + for the On Player Enter event and choose the PlayerHealth script and run the IncreaseHealth function.
I of course also added a spinning animation which pretty much has the exact same setup and steps as what I did in the https://sinevector241.itch.io/kit207-portfolio/devlog/705112/tutorial-3-kit207 dev log.
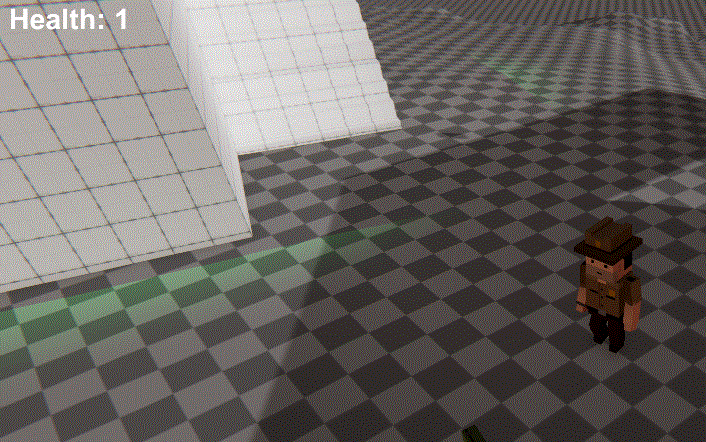
Last of all was adding an end, I didn't really want to put a massive amount of time into this so I spawned an energy explosion particle from the Particle Pack by Unity Technologies when the player health is 0 inside the PlayerController script.
void Update()
{
if(health.health <= 0)
{
gameObject.SetActive(false);
GameObject.Instantiate(particle, transform.position + new Vector3(0,2,0), Quaternion.identity);
return;
}
Result...
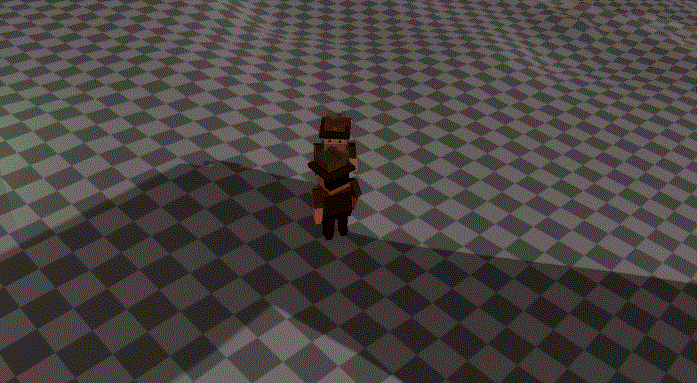
Simple Items by Synty - https://assetstore.unity.com/packages/3d/props/simple-items-cartoon-assets-36140
Particle Pack by Unity Technologies - https://assetstore.unity.com/packages/vfx/particles/particle-pack-127325
KIT207 Portfolio
Status | Released |
Category | Other |
Author | SineVector241 |
More posts
- Lighting, ShaderGraph and Tutorial 5 - KIT207Apr 16, 2024
- Game Ideas - KIT207Apr 07, 2024
- Tutorial 3 - KIT207Mar 28, 2024
- Terrain + ProBuilder - KIT207Mar 21, 2024
- Animation - KIT207Mar 17, 2024
- Tutorial 2 - KIT207Mar 15, 2024
- Tutorial 1 - KIT207Mar 15, 2024
- Blender Model - KIT207Mar 07, 2024
Leave a comment
Log in with itch.io to leave a comment.